My wife and I recently visited the V&A and stumbled across Chance and Control: Art in the age of computers, a small display celebrating 50 years of computer generated art. Tucked away in the corner of the second room was Schotter, a piece by Georg Nees.
“Nees was fascinated by the relationship between order and disorder in picture composition. To create this work, he introduced random variables into the computer program, causing orderly squares to descend into a heap.”
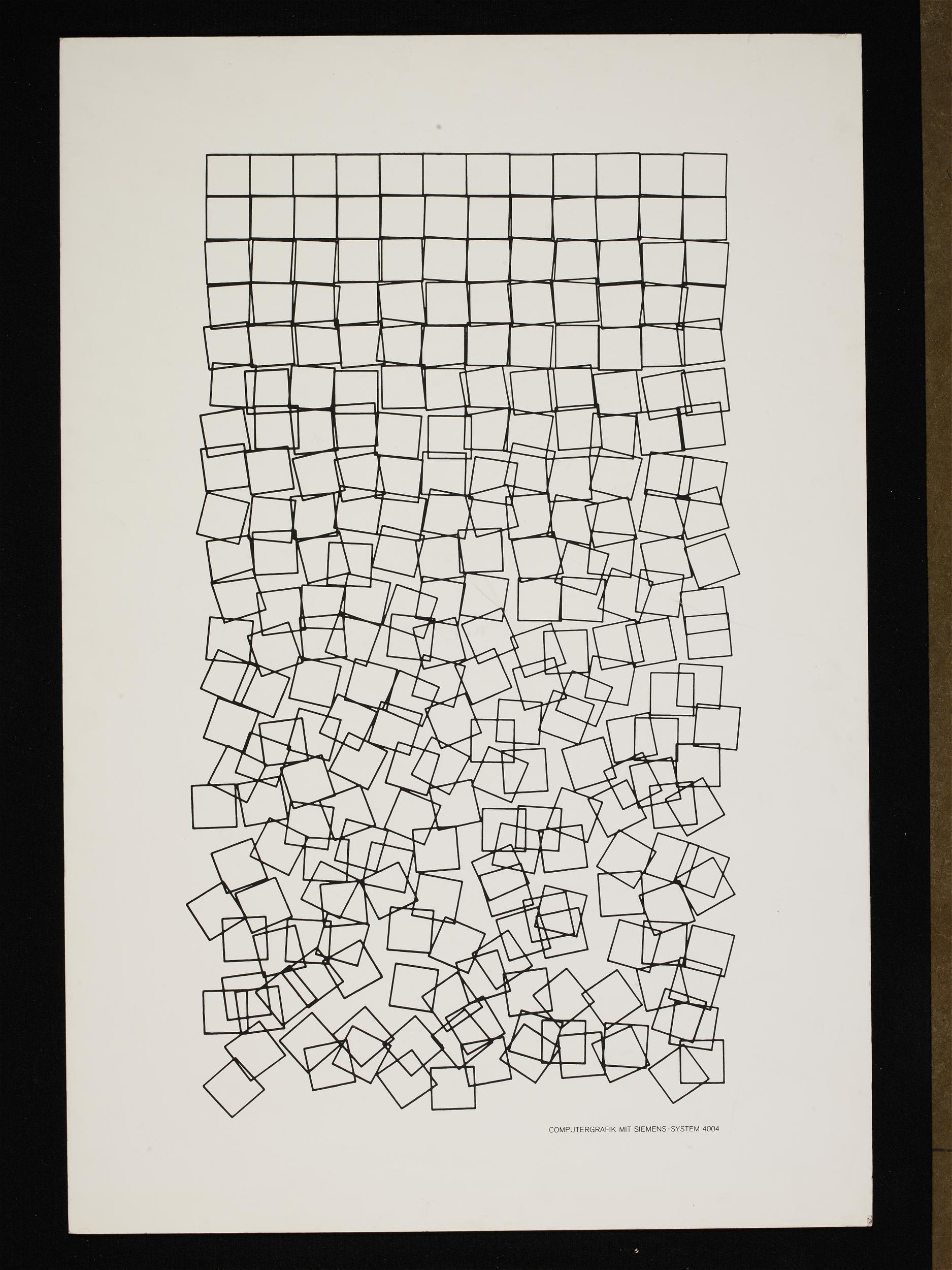
Schotter, Georg Nees
Schotter, Georg Nees© Victoria and Albert Museum, London
I was toying with the idea of recreating the image when I came across the Go Graphics package by Michael Fogleman (GitHub, GoDoc), a beautifully simple way to get started with drawing graphics in Go. The basics involve creating a context, drawing various shapes, stroking (or filling) them and then rendering the result as a PNG image.
package main
import "github.com/fogleman/gg"
func main() {
ctx := gg.NewContext(640, 640)
ctx.DrawRectangle(270, 270, 100, 100)
ctx.SetRGBA(0, 0, 0, 1)
ctx.Stroke()
ctx.SavePNG("out.png")
}
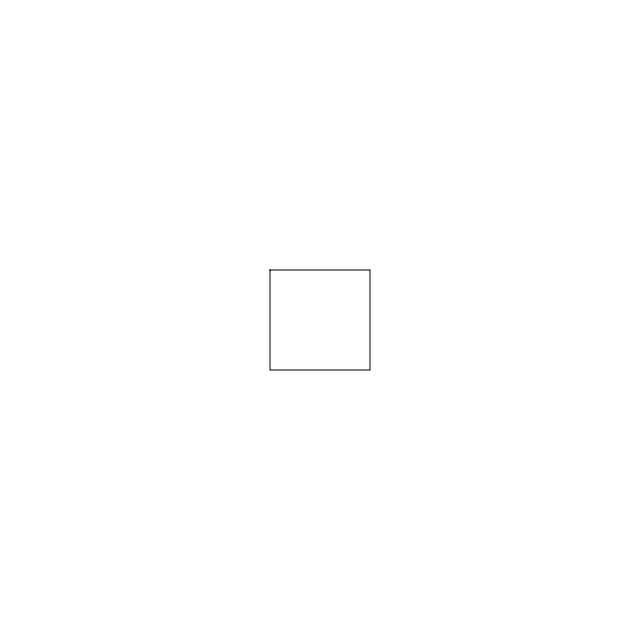
This code snippet creates a 640 by 640 image and draws a square of 100 by 100px in the center. To generate the image, I needed a few loops, some randomness and the ability to offset each square from it’s regular position. The algorithm seemed fairly straightforward:
- Use a
for
loop to iterate over the columns - Use a
for
loop to iterate over the rows - Offset each square from its mid-point based on a function of the current row
- Rotate each square based on a function of the current row
Tweaking the dimensions and weighting the offset and rotation gives us something remarkably similar to Georg’s original.
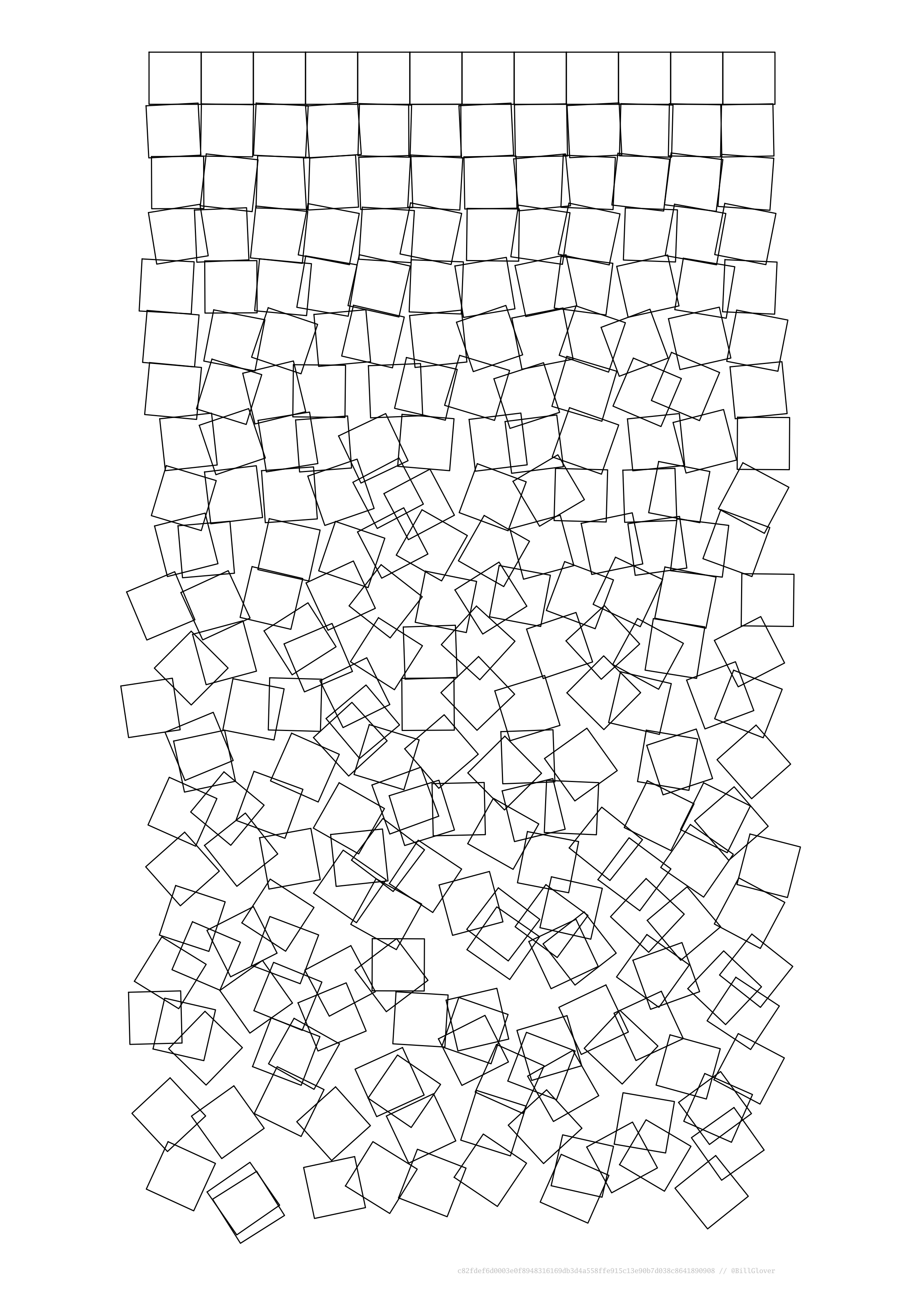
With the ability to produce images I quickly found myself spending hours tweaking parameters, padding, colours, etc. I settled on using an exponentially increasing random offset to generate the images below.
I’d love to try plotting some of these on paper (using a real plotter of course) but for now I’ll have to settle for generating wallpapers instead. A zip folder containing a collection of phone wallpapers is available for download below. Full access to the source code is available here: github.com/billglover/aitaoc